Today, numerous mobile and embedded applications utilize digital pencils for capturing signatures or similar tasks (such as in courier apps, bank offices, etc.). In this post, we’ll explore how to use PencilKit in SwiftUI.
Let’s start by defining our goal:
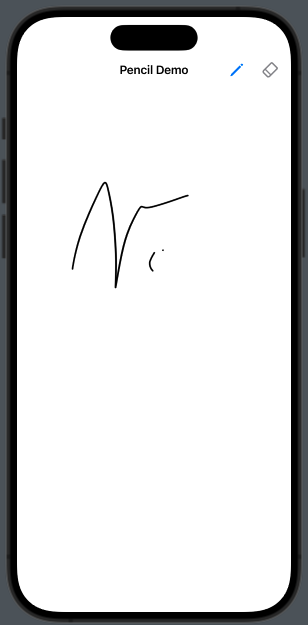
The ContentView define the settings of the applications:
import SwiftUI import PencilKit struct ContentView: View { @State var canvas = PKCanvasView() @State var drawing = true @State var color: Color = .black @State var type: PKInkingTool.InkType = .pen var body: some View { NavigationStack { CanvasView(canvas: $canvas, drawing: $drawing, type: $type) .navigationTitle("Pencil Demo") .font(.system(size: 35)) .navigationBarTitleDisplayMode(.inline) .foregroundStyle(Color.purple) .toolbar { Button(action: { drawing.toggle() }) { Image(systemName: "pencil") .font(.headline) .foregroundStyle(drawing ? Color.blue: Color.gray) } Button(action: { drawing.toggle() }) { Image(systemName: "eraser") .font(.headline) .foregroundStyle(drawing ? Color.gray: Color.blue) } } } } }
As we can see, here’s what we do:
- import the PencilKit
- Define the color
- Define the type of the tool (pencil or pen, etc.)
- Create a canvas of PKCanvasView() type
- Add two buttons to select what we want do, if draw or erase
- Call the CanvasView defined below
The CanvasView
struct CanvasView: UIViewRepresentable { @Binding var canvas: PKCanvasView @Binding var drawing: Bool @Binding var type: PKInkingTool.InkType var ink : PKInkingTool { PKInkingTool(type, color: UIColor(Color.black)) } var eraser = PKEraserTool(.bitmap) func makeUIView(context: Context) -> PKCanvasView { canvas.drawingPolicy = .anyInput canvas.tool = drawing ? ink : eraser return canvas } func updateUIView(_ uiView: PKCanvasView, context: Context) { uiView.tool = drawing ? ink : eraser } }
The CanvasView
implements the UIViewRepresentable
protocol, enabling us to incorporate a UIKit view within a SwiftUI. This approach allows for a seamless integration of PencilKit
functionalities into SwiftUI applications.
The variable ink
specifies the tool used on the canvas—be it a pen for drawing or an eraser for corrections. It’s worth noting the versatility of the eraser tool: it can be configured as a bitmap eraser, which erases only the specific parts it touches (optionally with a defined width), or as a vector eraser, which removes entire lines upon contact.
The makeUIView
method is responsible for initializing the PKCanvasView
, setting up the canvas where users can draw or write. On the other hand, the updateUIView
method ensures the canvas view is updated appropriately, reflecting any changes in its configuration or the tools used.
I encourage you to experiment with this virtual pen and explore the colors to truly understand the capabilities of this powerful tool. Dive into PencilKit
with SwiftUI and discover the potential of digital drawing and note-taking in your apps.